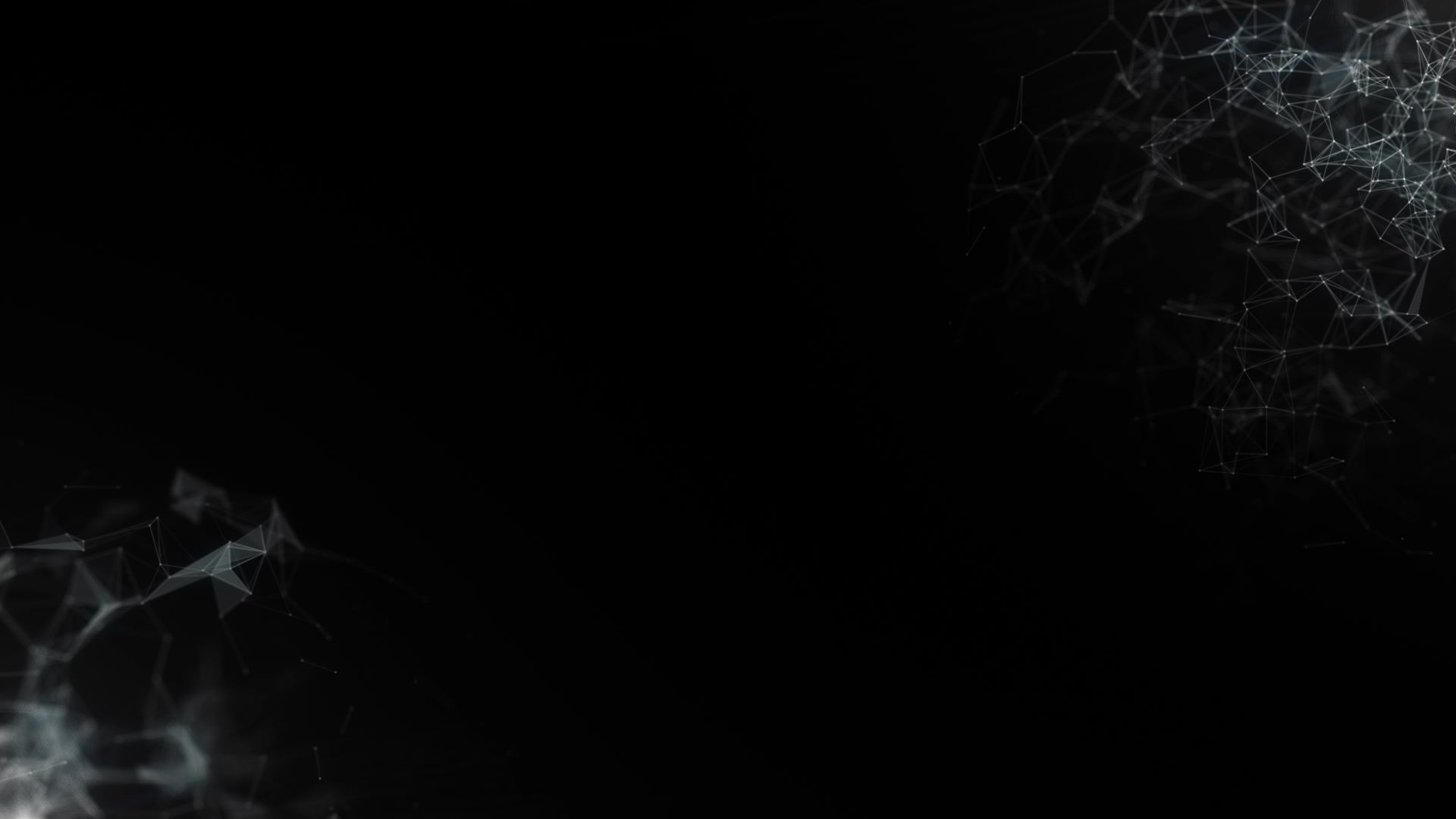
Mobile Game
c#
Character
Controller
using UnityEngine;
using System.Collections;
public class PlayerController : MonoBehaviour
{
public float moveSpeed;
public float attackTime;
public Vector2 LastMove;
public string startPoint;
public int deadZone = 50;
public float strongAttackDelay = 1.5f;
public float shieldDelay = 1.6f;
public GameObject touchControlsGUI;
public Camera camera;
private Animator anim;
private Rigidbody2D myRigidbody;
private bool playerMoving;
private bool attacking;
private float attackTimeCounter;
private int moveTouchId = -1;
private int attackTouchId = -1;
private Touch moveTouch;
private Touch attackTouch;
private float attackTouchStart;
private Vector2 moveTouchOrigin = Vector2.zero; //-Vector2.one;
private Vector2 attackTouchOrigin = Vector2.zero;
private Vector2 moveDirection = Vector2.zero;
private double midScreen;
private Transform dPad;
private Transform fingerTrack;
private Vector3 dPadPos;
public static int keyCount;
private static bool playerExists;
public bool canMove;
private SFXManager sfxMan;
// Use this for initialization
void Start()
{
sfxMan = FindObjectOfType<SFXManager>();
canMove = true;
dPad = touchControlsGUI.transform.GetChild(0);
moveTouchOrigin.x = 400;
moveTouchOrigin.y = 400;
dPadPos = camera.ScreenToWorldPoint (moveTouchOrigin);
dPadPos.z = 0f;
dPad.position = dPadPos;
dPad.GetComponent<Renderer>().enabled = true;
fingerTrack = touchControlsGUI.transform.GetChild(1);
//DontDestroyOnLoad(touchControlsGUI);
QualitySettings.vSyncCount = 0;
keyCount = 0;
midScreen = Screen.width * .5;
anim = GetComponent<Animator>();
myRigidbody = GetComponent<Rigidbody2D>();
if(!playerExists)
{
playerExists = true;
DontDestroyOnLoad(transform.gameObject);
} else {
Destroy(gameObject);
}
}
// Fixed update is called after physics is calculated
void FixedUpdate ()
{
if(!canMove)
{
myRigidbody.velocity = Vector2.zero;
return;
}
if (playerMoving) {
myRigidbody.velocity = moveDirection.normalized * moveSpeed; // Normalize so diagonal movement isn't faster
//Debug.Log("[RLSL] moveDirection: " + moveDirection + " Time.deltaTime: " + Time.deltaTime + " Velocity: " + myRigidbody.velocity);
} else {
myRigidbody.velocity = Vector2.zero;
}
}
// Update is called once per frame
void Update() {
float lerpFactor = (Time.time - Time.fixedTime)/Time.fixedDeltaTime;
transform.position = myRigidbody.position + ( myRigidbody.velocity * Time.fixedDeltaTime*lerpFactor );
// Keyboard controls
#if UNITY_STANDALONE || UNITY_WEBPLAYER || UNITY_EDITOR
moveDirection.x = Input.GetAxis ("Horizontal");
moveDirection.y = Input.GetAxis ("Vertical");
if (Input.GetKeyDown(KeyCode.Space))
{
attackTimeCounter = attackTime;
attacking = true;
anim.SetBool("Attack", true);
sfxMan.playerAttack.Play();
}
// Touch controls
#elif UNITY_IOS || UNITY_ANDROID || UNITY_WP8 || UNITY_IPHONE
if (Input.touchCount > 0 || attackTouchId != -1 || moveTouchId != -1) {
foreach(Touch touch in Input.touches) {
// Find existing touches
if (touch.fingerId == moveTouchId) {
moveTouch = touch;
} else if (touch.fingerId == attackTouchId) {
attackTouch = touch;
}
// Assign new touches
else {
if (touch.position.x < midScreen) { // Left side
if (moveTouchId == -1) { // Only use the first touch on the left for move
moveTouchId = touch.fingerId;
moveTouch = touch;
}
} else { // Right side
if (attackTouchId == -1) { // Only use the first touch on the right for attack
attackTouchId = touch.fingerId;
attackTouch = touch;
}
}
}
}
// Move
if (moveTouchId != -1) {
if (moveTouch.phase == TouchPhase.Began) {
/*moveTouchOrigin = moveTouch.position;
Vector3 dPadPos = camera.ScreenToWorldPoint (moveTouchOrigin);
dPadPos.z = 0f;
dPad.position = dPadPos;
dPad.GetComponent<Renderer>().enabled = true;
*/
fingerTrack.position = dPadPos;
fingerTrack.GetComponent<Renderer>().enabled = true;
} else if (moveTouch.phase == TouchPhase.Moved) {
moveDirection = Vector2.zero;
Vector2 touchPos = moveTouch.position;
float x = touchPos.x - moveTouchOrigin.x;
float y = touchPos.y - moveTouchOrigin.y;
Vector3 fingerTrackPos = camera.ScreenToWorldPoint (touchPos);
fingerTrackPos.z = 0f;
fingerTrack.position = fingerTrackPos;
if (x < -deadZone) {
moveDirection += Vector2.left;
} else if (x > deadZone) {
moveDirection += Vector2.right;
}
if (y < -deadZone) {
moveDirection += Vector2.down;
} else if (y > deadZone) {
moveDirection += Vector2.up;
}
} else if (moveTouch.phase == TouchPhase.Ended || moveTouch.phase == TouchPhase.Canceled) {
//dPad.GetComponent<Renderer>().enabled = false;
fingerTrack.GetComponent<Renderer>().enabled = false;
moveDirection = Vector2.zero;
moveTouchId = -1;
}
}
// Attack
if (attackTouchId != -1) {
if (attackTouch.phase == TouchPhase.Began) {
attackTouchOrigin = attackTouch.position;
attackTouchStart = Time.realtimeSinceStartup;
if (!attacking) {
attacking = true;
attackTimeCounter = attackTime;
anim.SetBool("Attack", true);
sfxMan.playerAttack.Play();
}
} else if (attackTouch.phase == TouchPhase.Moved || attackTouch.phase == TouchPhase.Stationary) {
float attackTouchHold = Time.realtimeSinceStartup - attackTouchStart;
if (attackTouchHold > shieldDelay) {
Debug.Log("[RLSL] Activate shield");
}
} else if (attackTouch.phase == TouchPhase.Ended || attackTouch.phase == TouchPhase.Canceled) {
float attackTouchEnd = Time.realtimeSinceStartup - attackTouchStart;
Vector2 touchPos = attackTouch.position;
float x = touchPos.x - attackTouchOrigin.x;
float y = touchPos.y - attackTouchOrigin.y;
if (attackTouchEnd > strongAttackDelay) {
Debug.Log("[RLSL] Strong attack");
if (attackTouchEnd > shieldDelay) {
Debug.Log("[RLSL] Deactivate shield");
}
} else {
if(Mathf.Abs(x) >= Mathf.Abs(y)) {
if (x < -deadZone) { // Left swipe
Debug.Log("[RLSL] Left swipe");
} else if (x > deadZone) { // Right swipe
Debug.Log("[RLSL] Right swipe");
}
} else {
if (y < -deadZone) { // Down swipe
Debug.Log("[RLSL] Down swipe");
} else if (y > deadZone) { // Up swipe
Debug.Log("[RLSL] Up swipe");
}
}
}
attackTouchId = -1;
}
}
}
#endif
if (moveDirection != Vector2.zero) {
playerMoving = true;
LastMove = moveDirection;
anim.SetFloat("MoveX", moveDirection.x);
anim.SetFloat("MoveY", moveDirection.y);
anim.SetFloat("LastMoveX", LastMove.x);
anim.SetFloat("LastMoveY", LastMove.y);
} else {
playerMoving = false;
}
anim.SetBool("PlayerMoving", playerMoving);
if(attackTimeCounter > 0) {
attackTimeCounter -= Time.deltaTime;
} else {
attacking = false;
anim.SetBool("Attack", false);
}
}
public void Stop() {
dPad.GetComponent<Renderer>().enabled = false;
fingerTrack.GetComponent<Renderer>().enabled = false;
moveDirection = Vector2.zero;
moveTouchId = -1;
playerMoving = false;
anim.SetBool("PlayerMoving", playerMoving);
GetComponent<Rigidbody2D>().velocity = Vector2.zero;
}
}